using System;
using WinSCP;
class Example
{
public static int Main()
{
try
{
// Setup session options
SessionOptions sessionOptions = new SessionOptions
{
Protocol = Protocol.Sftp,
HostName = "example.com",
UserName = "user",
Password = "mypassword",
SshHostKeyFingerprint = "ssh-rsa 2048 xxxxxxxxxxx...="
};
using (Session session = new Session())
{
// Connect
session.Open(sessionOptions);
// Upload files
TransferOptions transferOptions = new TransferOptions();
transferOptions.TransferMode = TransferMode.Binary;
TransferOperationResult transferResult;
transferResult =
session.PutFiles(@"d:\toupload\*", "/home/user/", false, transferOptions);
// Throw on any error
transferResult.Check();
// Print results
foreach (TransferEventArgs transfer in transferResult.Transfers)
{
Console.WriteLine("Upload of {0} succeeded", transfer.FileName);
}
}
return 0;
}
catch (Exception e)
{
Console.WriteLine("Error: {0}", e);
return 1;
}
}
}
public string[] GetFileList()
{
List<string> list = new List<string>();
try
{
// Create a new Sftp instance.
SessionOptions sessionOptions = new SessionOptions
{
Protocol = Protocol.Sftp,
HostName = ServerAddress,
UserName = OutUser,
Password = OutPwd,
//SshHostKeyFingerprint = "ssh-rsa 2048 xx:xx:xx:xx:xx:xx:xx:xx..."
GiveUpSecurityAndAcceptAnySshHostKey = true,
};
using (Session session = new Session())
{
// Connect
session.Open(sessionOptions);
// Enumerate files and directories to download
list = session.EnumerateRemoteFiles(OutDirectory, null, EnumerationOptions.None)
.Select(f => f.Name).ToList();
}
return list.ToArray();
}
catch (Exception ex)
{
log.ErrorFormat("GetFtpFileList: {0}", ex.Message);
return null;
}
}
Note: If ppk is required by destination FTP site, make sure the ppk is loaded into Pageant first. Else authentication will fail when the .NET app tries to connect to the FTP site, even if “GiveUpSecurityAndAcceptAnySshHostKey = true” is set.
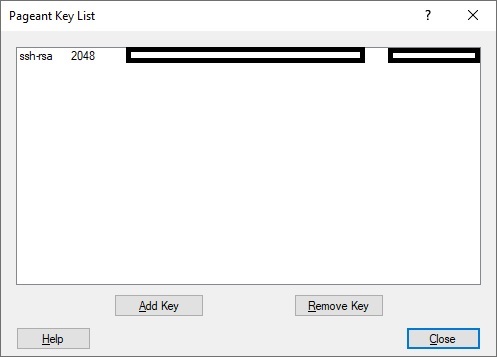
Comments